https://loy124.tistory.com/204 (s3 서버 구축하기)
https://loy124.tistory.com/205?category=768865 (s3 CloudFront와 연동하기)
기초 셋팅
https://loy124.tistory.com/206
백엔드 구성편
https://loy124.tistory.com/207
프론트 구성편
https://loy124.tistory.com/208
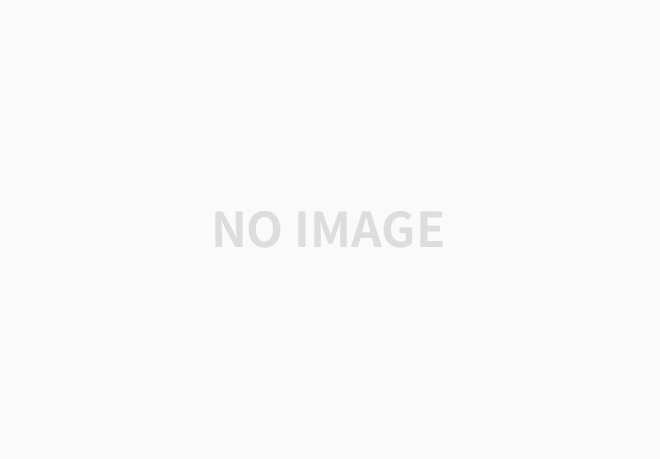
gallery.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<!-- bit.com.a.dao.BbsDao -->
<mapper namespace="bit.com.a.gallery.GalleryDao">
<insert id="upload" parameterType="bit.com.a.gallery.GalleryDto" useGeneratedKeys="true" keyProperty="id">
INSERT INTO gallery(title, file_path) VALUES(#{title}, #{filePath})
</insert>
<select id="getImageList" resultType="bit.com.a.gallery.GalleryDto">
SELECT file_path, title from gallery
</select>
<!-- <select id="updateImage" parameterType="bit.com.a.gallery.GalleryDto">
Update gallery set where
</select> -->
</mapper>
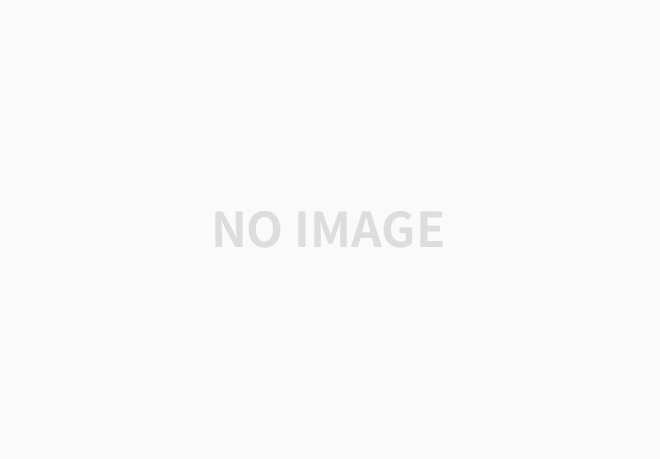
S3Service.java (aws S3에접속하여 해당 서비스에서 실제 파일을 업로드한다)
실제 파일은 S3에 올라가고
db에는 파일의 주소가 올라가는 방식이다
@value는 application.properties에서 값을 가져온다.
package bit.com.a.s3;
import java.io.IOException;
import java.time.LocalDateTime;
import javax.annotation.PostConstruct;
import com.amazonaws.auth.AWSCredentials;
import com.amazonaws.auth.AWSStaticCredentialsProvider;
import com.amazonaws.auth.BasicAWSCredentials;
import com.amazonaws.services.s3.AmazonS3;
import com.amazonaws.services.s3.AmazonS3ClientBuilder;
import com.amazonaws.services.s3.model.CannedAccessControlList;
import com.amazonaws.services.s3.model.PutObjectRequest;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Service;
import org.springframework.web.multipart.MultipartFile;
/**
* S3Service
*/
@Service
public class S3Service {
public static final String CLOUD_FRONT_DOMAIN_NAME = "https://" + "CLOUDFRONT의 주소" + "/";
private AmazonS3 s3Client;
@Value("${cloud.aws.credentials.accessKey}")
private String accessKey;
@Value("${cloud.aws.credentials.secretKey}")
private String secretKey;
@Value("${cloud.aws.s3.bucket}")
private String bucket;
@Value("${cloud.aws.region.static}")
private String region;
public S3Service() {
}
@PostConstruct
public void setS3Client() {
AWSCredentials credentials = new BasicAWSCredentials(this.accessKey, this.secretKey);
s3Client = AmazonS3ClientBuilder.standard().withCredentials(new AWSStaticCredentialsProvider(credentials))
.withRegion(this.region).build();
}
public String upload(MultipartFile file) throws IOException {
String fileName = file.getOriginalFilename();
s3Client.putObject(new PutObjectRequest(bucket, fileName, file.getInputStream(), null)
.withCannedAcl(CannedAccessControlList.PublicRead));
return fileName;
}
public String upload(String currentFilePath, MultipartFile file) throws IOException {
// 고유한 key 값을 갖기위해 현재 시간을 postfix로 붙여줌
// SimpleDateFormat date = new SimpleDateFormat("yyyymmddHHmmss");
String fileName = file.getOriginalFilename() + "-" + LocalDateTime.now();
// + date.format(new Date());
// key가 존재하면 기존 파일은 삭제
if ("".equals(currentFilePath) == false && currentFilePath != null) {
boolean isExistObject = s3Client.doesObjectExist(bucket, currentFilePath);
if (isExistObject == true) {
s3Client.deleteObject(bucket, currentFilePath);
}
}
// 파일 업로드
s3Client.putObject(new PutObjectRequest(bucket, fileName, file.getInputStream(), null)
.withCannedAcl(CannedAccessControlList.PublicRead));
return fileName;
}
}
dto 생성하기
GalleryDto.java
package bit.com.a.gallery;
public class GalleryDto {
private Long id;
private String title;
private String filePath;
private String imgFullPath;
public GalleryDto() {
}
public GalleryDto(Long id, String title, String filePath, String imgFullPath) {
this.id = id;
this.title = title;
this.filePath = filePath;
this.imgFullPath = imgFullPath;
}
@Override
public String toString() {
return "{" + " id='" + id + "'" + ", title='" + title + "'" + ", filePath='" + filePath + "'" + ", imgFullPath='"
+ imgFullPath + "'" + "}";
}
public Long getId() {
return this.id;
}
public void setId(Long id) {
this.id = id;
}
public String getTitle() {
return this.title;
}
public void setTitle(String title) {
this.title = title;
}
public String getFilePath() {
return this.filePath;
}
public void setFilePath(String filePath) {
this.filePath = filePath;
}
public String getImgFullPath() {
return this.imgFullPath;
}
public void setImgFullPath(String imgFullPath) {
this.imgFullPath = imgFullPath;
}
}
GalleryDao.java
package bit.com.a.gallery;
import java.util.List;
import org.apache.ibatis.annotations.Mapper;
import org.springframework.stereotype.Repository;
@Mapper
@Repository
public interface GalleryDao {
public int upload(GalleryDto galleryDto);
public List<GalleryDto> getImageList();
}
GalleryService.java
package bit.com.a.gallery;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
/**
* GalleryService
*/
@Service
@Transactional
public class GalleryService {
@Autowired
GalleryDao galleryDao;
public int upload(GalleryDto galleryDto) {
System.out.println("dao");
System.out.println(galleryDto.toString());
return galleryDao.upload(galleryDto);
}
public List<GalleryDto> getImageList() {
return galleryDao.getImageList();
}
}
GalleryController.java
package bit.com.a.gallery;
import java.io.IOException;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import bit.com.a.s3.S3Service;
/**
* GalleryController
*/
@RestController
@RequestMapping("/api/gallery")
public class GalleryController {
//
@Autowired
private GalleryService galleryService;
@Autowired
private S3Service s3Service;
@PostMapping("/upload")
public String execWrite(GalleryDto galleryDto, MultipartFile files) throws IOException {
System.out.println(files);
// for (int i = 0; i < files.length; i++) {
String imgPath = s3Service.upload(galleryDto.getFilePath(), files);
galleryDto.setFilePath("https://" + "dixo0q5vi6g16.cloudfront.net" + "/" + imgPath);
// }
return galleryService.upload(galleryDto) > 0 ? "OK" : "FAIL";
}
@GetMapping("/getImageList")
public List<GalleryDto> getImageList() {
return galleryService.getImageList();
}
}
백엔드 구성 완료
'Java > Spring-boot' 카테고리의 다른 글
Spring boot AOP를 활용한 로그 추적하기, 메서드 시간 측정하기 (0) | 2023.07.07 |
---|---|
AOP정의 및 Advice, Pointcut 작성 정리 (0) | 2023.07.06 |
Spring-boot(mysql-mybatis-aws s3(cloudFront) + Vue.js 를 활용한 파일 업로드 하기 - 기초 셋팅 (0) | 2020.04.12 |
이클립스(스프링 sts)에서 Spring-boot, Jpa 사용 하기 (2) (0) | 2020.03.15 |
이클립스(스프링 sts)에서 Spring-boot, Jpa 사용 하기 (1) (0) | 2020.03.15 |